Algorithms and Data Structures for Programming Contests
Graph Traversal Algorithms
Discussion
What is this about?
- Graphs G = (V,E):
- Vertex (or node) set V =
{v1,...,vn}.
- Edge (or arc) set E =
{e1,...,em}, where
ej = (u,v)
for u,v ∈ V.
- Directed graph:
(u,v) ≠ (v,u),
self-loops (v,v) allowed, e.g.,
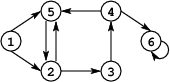
- Undirected graph:
(u,v) = (v,u),
self-loops disallowed, e.g.,
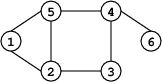
- Terminology: u is neighbour of v
if (v,u) ∈ E — note: different from
(u,v) ∈ E.
- Traversal algorithm:
systematic way to "visit" each vertex in G.
Two standard traversals:
depth-first search (DFS),
breadth-first search (BFS).
Depth-first search
- Given graph G = (V,E)
and first node to visit.
- Initialization:
- Mark each node "undiscovered".
- Push first node onto empty stack.
- While stack not empty:
- Remove top node v from stack.
- If v is "discovered", go back to start of loop.
- Mark v "discovered".
- Visit v (application-specific).
- Push all undiscovered neighbours of v onto stack.
Breadth-first search
- Given graph G = (V,E)
and first node to visit.
- Initialization:
- Mark each node "undiscovered".
- Append first node to empty queue.
- While queue not empty:
- Remove front node v from queue.
- If v is "discovered", go back to start of loop.
- Mark v "discovered".
- Visit v (application-specific).
- Append all undiscovered neighbours of v to queue.
Try it! (1)
- Try the
"Portals Redux"
problem from the DWITE programming contest.
- Ignore issues of input/output for now:
suppose you already have the data stored
in an appropriate data structure of your choice.
© Copyright 2012–2015 François Pitt
<francois.pitt@utoronto.ca>
last updated at 08:25 (EST) on Thu 5 Mar 2015
Algorithms and Data Structures by
François Pitt is licensed under a
Creative
Commons Attribution-ShareAlike 4.0 International License.